Are you interested in building a digital clock using Python and PySide2? Look no further! In this article, we'll explore how to create a simple yet functional GUI digital clock using PySide2's QLCDNumber and QTimer classes. We'll also provide a brief overview of the YouTube tutorial that inspired this project.
Introduction to PySide2
PySide2 is a Python binding for the Qt application framework, which is written in C++. It's a popular choice for building GUI applications due to its ease of use, flexibility, and cross-platform compatibility. With PySide2, you can create complex and visually appealing GUI applications with minimal code.
QLCDNumber and QTimer: The Building Blocks of Our Digital Clock
To build our digital clock, we'll be using two essential classes from PySide2: QLCDNumber and QTimer.
QLCDNumber: This class is used to display numerical values in a LCD-like format. It's perfect for creating a digital clock display.
QTimer: This class is used to create timers that can be used to trigger events at regular intervals. In our case, we'll use it to update the time display every second.
Building the Digital Clock
To build the digital clock, follow these steps:
1. Import the necessary modules, including PySide2 and sys.
2. Create a QApplication instance to initialize the GUI event loop.
3. Create a QWidget instance to serve as the main window for our application.
4. Create a QLCDNumber instance to display the time.
5. Create a QTimer instance to update the time display every second.
6. Connect the QTimer's timeout signal to a slot function that updates the time display.
7. Show the main window and start the GUI event loop.
Example Code
Here's some example code to get you started:
```python
import sys
from PySide2.QtCore import QTimer
from PySide2.QtWidgets import QApplication, QWidget, QLCDNumber, QVBoxLayout
class DigitalClock(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.lcd = QLCDNumber()
self.timer = QTimer()
self.timer.timeout.connect(self.showTime)
self.timer.start(1000)
layout = QVBoxLayout()
layout.addWidget(self.lcd)
self.setLayout(layout)
self.showTime()
self.show()
def showTime(self):
import time
currentTime = time.strftime("%H:%M:%S")
self.lcd.display(currentTime)
def main():
app = QApplication(sys.argv)
clock = DigitalClock()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
```
This code creates a simple digital clock that displays the current time in hours, minutes, and seconds.
In this article, we explored how to create a Python GUI digital clock using PySide2's QLCDNumber and QTimer classes. We also provided a brief overview of the YouTube tutorial that inspired this project. With this knowledge, you can create your own digital clock application using PySide2. So why not give it a try? Download the PySide2 library, follow the steps outlined in this article, and start building your own digital clock today!
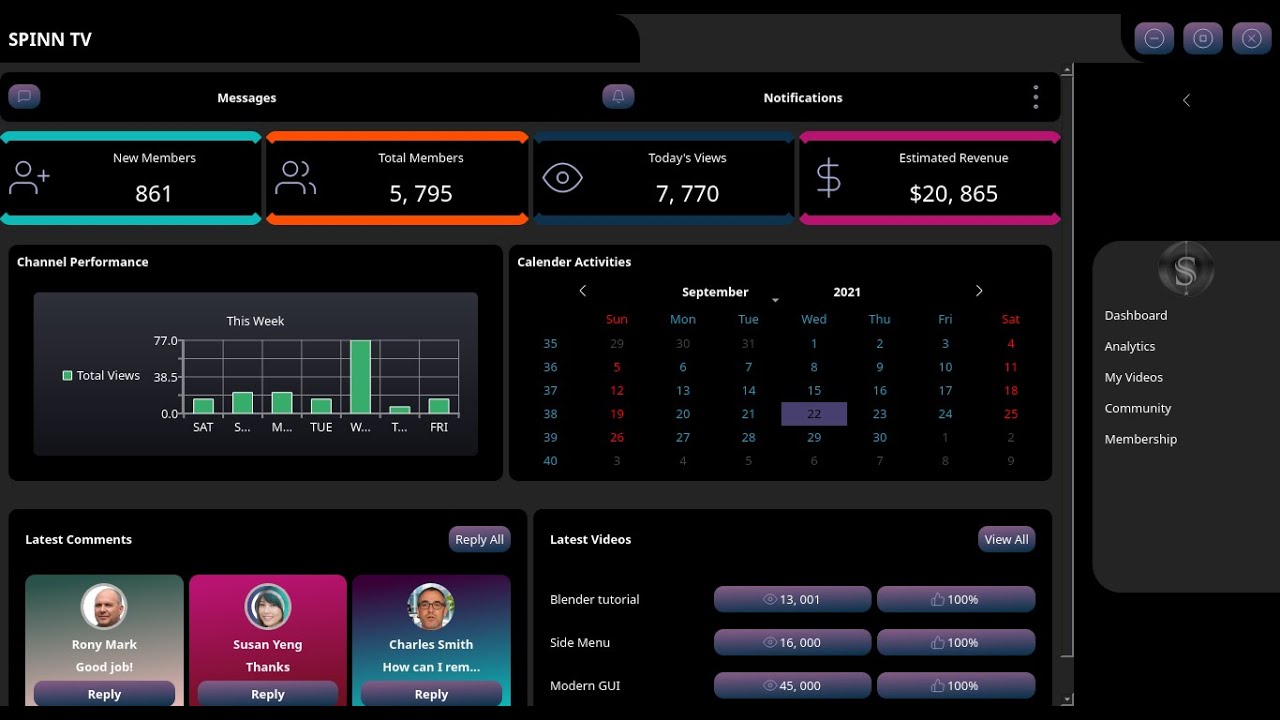
Watch the YouTube Tutorial
If you're interested in learning more about this project, be sure to check out the
YouTube tutorial that inspired this article. The tutorial provides a step-by-step guide on how to create a digital clock using PySide2, including how to use QLCDNumber and QTimer.
By following this tutorial and practicing with the example code provided, you'll be well on your way to creating your own GUI applications using PySide2. Happy coding!